How to Create a SearchStax Custom Search Page
In a real-world project, customers using the SearchStax Sitecore Module to leverage all the SearchStudio capabilities will end up needing to customize the Sitecore search page in order to give it the same look and feel of the rest of the website, or to extend some missing feature. Instead of editing the SearchStudio search page component, the best way to do that is by creating a custom search page.
Do not modify SearchStax components!
SearchStax strongly recommends that code customization should not be performed on the original SearchStax Sitecore components. Any change applied to an out-of-the-box component might be overwritten during an upgrade process. To avoid this situation, you should create a new component using the original search page as starting point and then apply any customization needed.
This tutorial is a step by step that shows how to create a full custom component.
Prerequisites
This article assumes that you already have a C# solution with an MVC project.
Assembly References
Start by adding project references to those two SearchStax assemblies. They are part of your SearchStax for Sitecore package:
- SearchStax.Foundation.Core.dll
- SearchStax.Feature.SearchPage.dll
Controller
Add the below class to your MVC project and give it a meaningful name following the MVC pattern, such as CustomSearchStaxController. This class will have an Index() action method that will be used by a Sitecore controller rendering later. This method loads everything required by a SearchStax view class to work properly and passes it to the view using the SearchPageModel object.
using SearchStax.Feature.SearchPage.Services;
using System.Web.Mvc;
using System.IO;
using System;
namespace SearchStax.Feature.SearchPage.Controllers
{
public class CustomSearchStaxController : Controller
{
private readonly ISearchPageConfigurationFetcher _searchPageConfigurationFetcher;
public CustomSearchStaxController()
: this(new SearchPageConfigurationFetcher())
{ }
public CustomSearchStaxController(ISearchPageConfigurationFetcher searchPageConfigurationFetcher)
{
this._searchPageConfigurationFetcher = searchPageConfigurationFetcher;
}
public ActionResult Index()
{
var searchModel = this._searchPageConfigurationFetcher.GetSearchPageConfiguration();
string viewName = "Index";
var viewFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "Views\\SearchStaxPage\\" + searchModel.SearchStaxConfiguration.IndexCoreName + ".cshtml");
if (System.IO.File.Exists(viewFilePath))
{
viewName = searchModel.SearchStaxConfiguration.IndexCoreName;
}
return View(viewName, searchModel);
}
}
}
View
Create a new view file based on the already existing \Views\SearchStaxPage\Index.cshtml file found on your Sitecore instance. Name this file as Index.cshtml and add it to the project folder \Views\[CustomSearchStax]. Make sure you change the [CustomSearchStax] by your controller prefix.
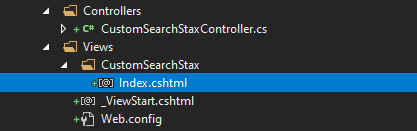
This is the moment when you can apply some custom styling with CSS manipulations, or even more structural changes to the search page, accordingly to what is required by your project design.
Rendering
In Sitecore, create a controller rendering under a custom folder on /sitecore/layout/Renderings. Configure the Controller field to point to the previously created controller, and the Controller Action field to its Index method. Here is an example:
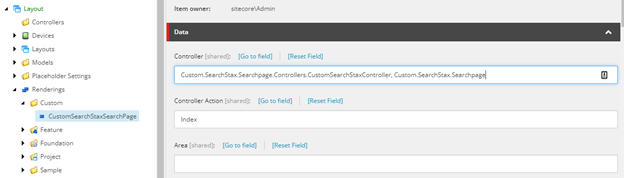
Search Page
Once you have everything configured in the rendering, you can add the new rendering to a page item and validate that the customizations you previously applied are there.
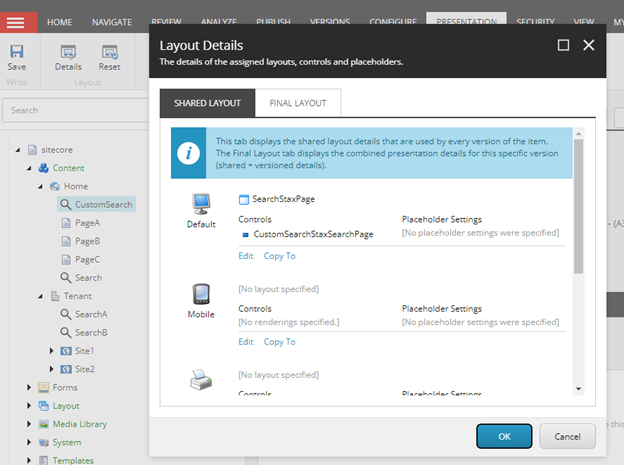
You can even compare this search page with the standard one that uses the standard /sitecore/layout/Renderings/Feature/SearchStax/SearchPage/SearchStaxPageRendering to see the customizations:
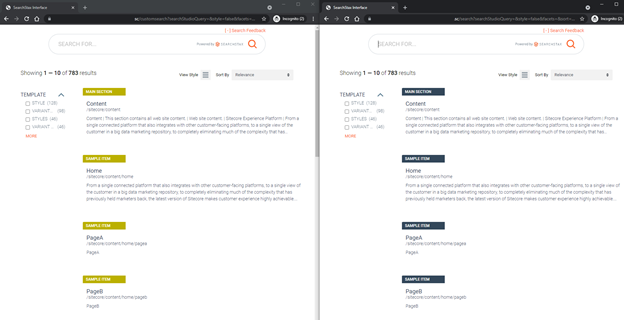
Summary
- Chose an MVC project or create a new one.
- Add a reference to SearchStax.Foundation.Core.dll
- Add a reference to SearchStax.Feature.SearchPage.dll
- Create a new controller called CustomSearchStaxController
- Copy and paste the controller class from the example
- Create a new view based on \Views\SearchStaxPage\Index.cshtml and add it to \Views\ CustomSearchStax\Index.cshtml
- Create a new rendering pointing to the method in the new controller
- Add the rendering to a search page and validate the customizations
Questions?
Do not hesitate to contact the SearchStax Support Desk.